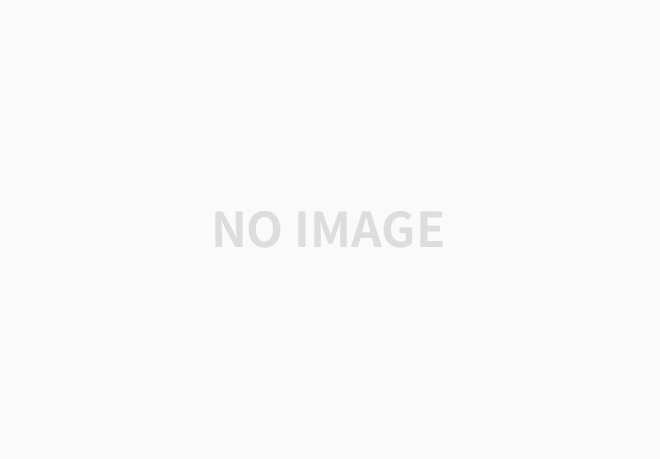
cocos2d-x를 한번 사용해보자
우선 cocos2d-x파일을 설치 및 자동 환경설정을 하기 위해서는 홈페이지에서 zip파일을 다운받은 다음 setup.py를 실행시켜야한다. 이를 위해 python을 설치해주자.
검색해봤을 때 python 버전은 2버전대를 설치해주자
이 버전을 설치하는 이유는 cocos2d-console 도구가 Python 2 문법 기반으로 작성되어있기 때문이다.
https://www.python.org/downloads/release/python-2718/
Python Release Python 2.7.18
The official home of the Python Programming Language
www.python.org
이 링크에 접속한 다음 자신의 컴퓨터에 맞는 실행파일을 다운 받아 설치해주면된다. 설치할 때 add to path를 추가해주어야 정상적으로 작동한다.
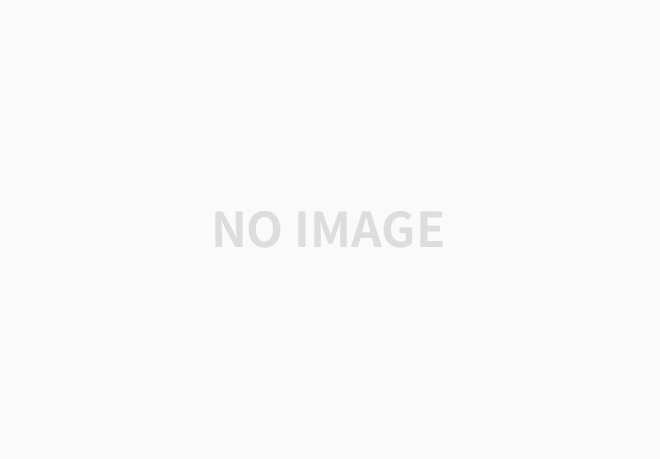
이렇게 설치해준 다음, cocos2d-x 파일을 다운받아주자. cocos2dx는 버전은 3.17.2로 진행하였다.
https://www.cocos.com/en/cocos2dx-download
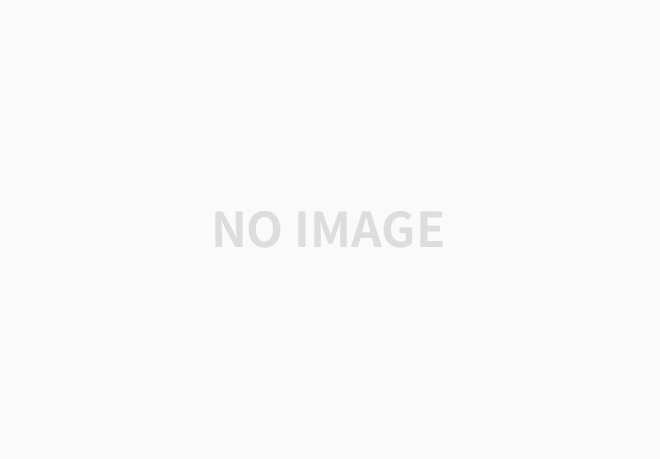
이렇게 다운 받은 다음 cmd창이나 bash창을 통해 cocos2d-x파일의 압축을 푼 경로로 이동하여 다음 명령어를 입력해주자.
python setup.py
이렇게 해주면 입력하라는 창이 나올텐데 이것은 SDK 경로를 입력하는 창으로 당장은 필요하지 않기 때문에 Enter를 눌러 넘어가주자.
이렇게 해준다음 cocos 명령어를 통해 프로젝트를 생성하면 된다. 명령어는 위와 동일하게 cmd창이나 bash창에 입력하면 된다. 중요한 점은 이때 프로젝트는 cocos2d-x의 파일을 압축푼 곳에 생성해야 올바르게 작동한다는 점이다. 나는 projects라는 새로운 폴더를 만들고 이곳에 프로젝트를 생성해주었다.
C:\cocos2d-x-3.17.2\projects
cocos new [프로젝트 이름] -p [패키지 이름] -l [언어] -d [경로]
이렇게 만들어준 다음 proj.win32 폴더로 가서 sln파일을 열어주고 실행해보았을 때 cocos2d-x 아이콘과 화면이 등장하면 성공이다.
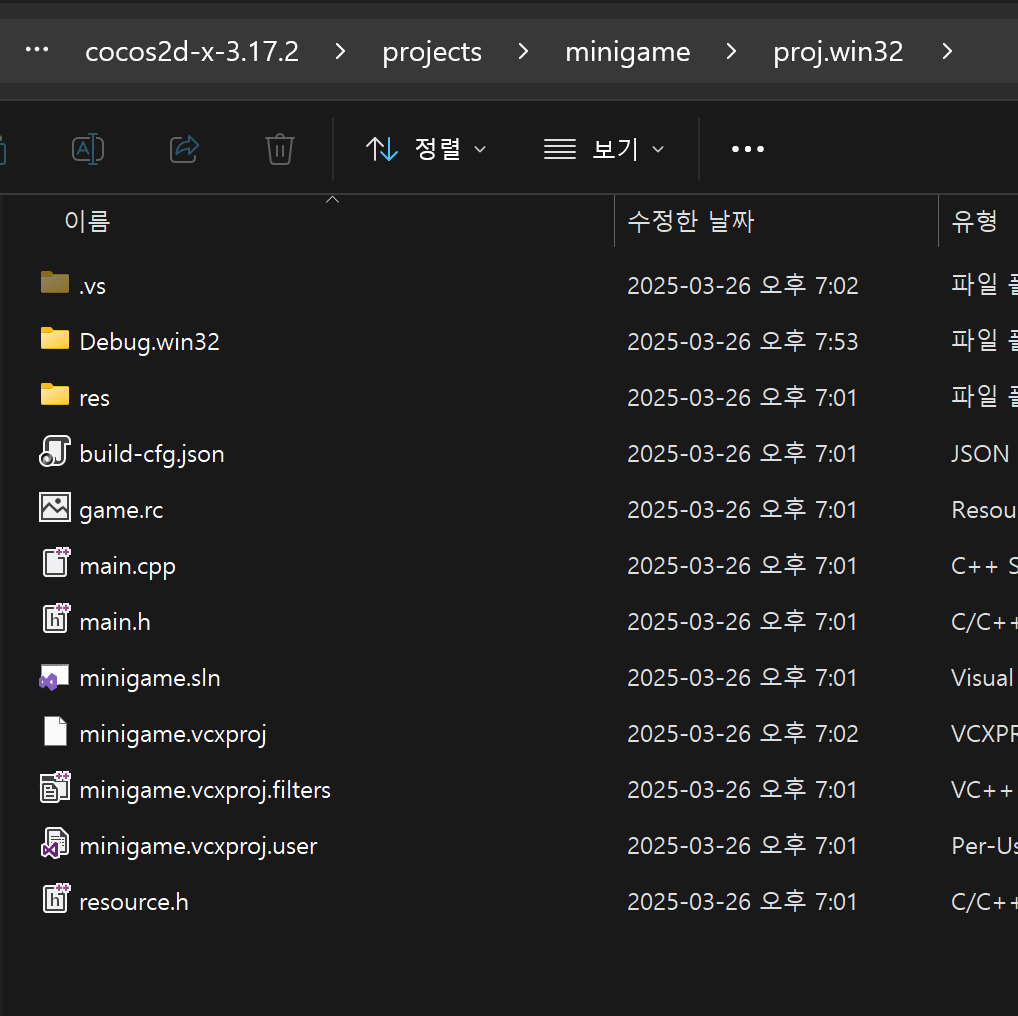
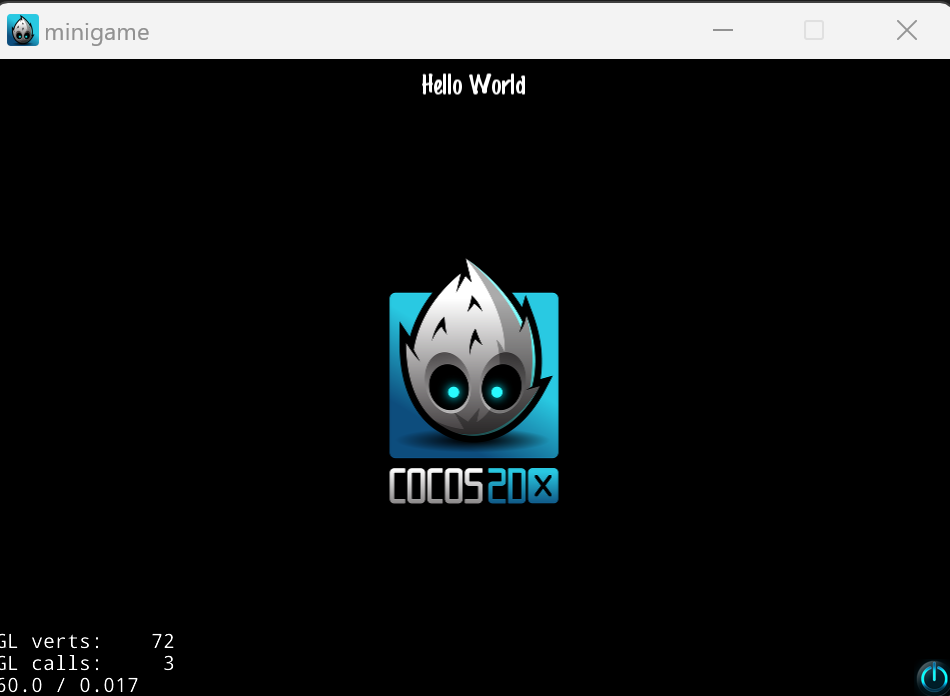